
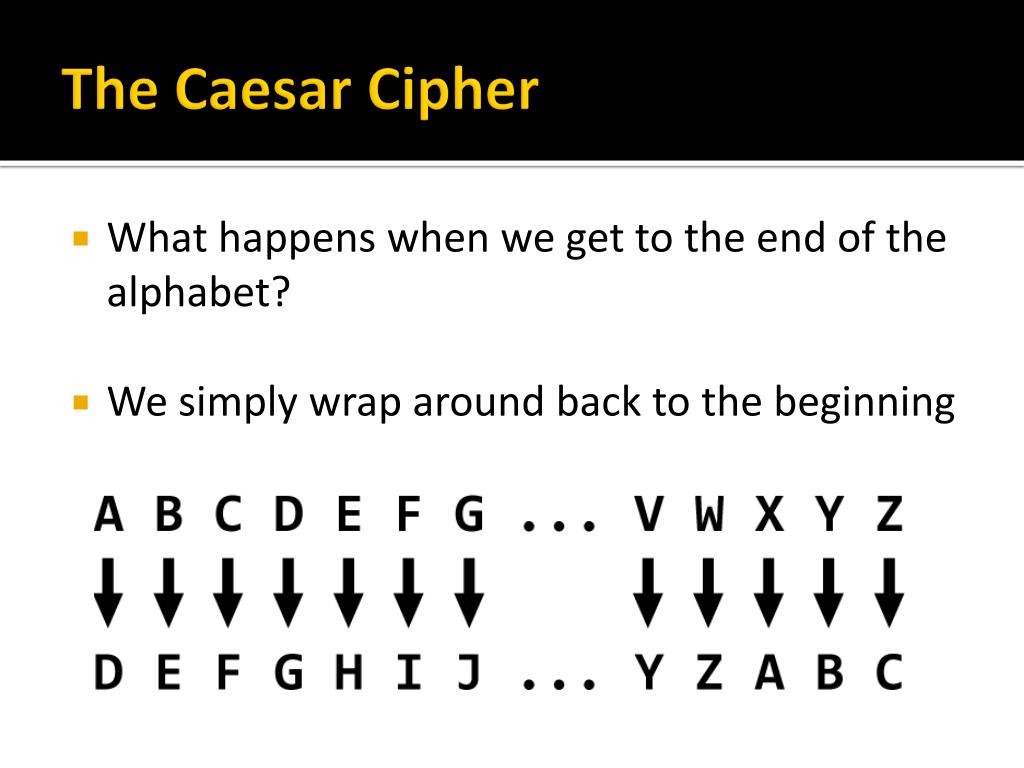
Since the whole point of this exercise is to test drive awesome languages, I’m going to implement the algorithm in Haskell, a purely functional language. For example, the relative frequencies of the string “AB” in alphabetical order are 0.5, 0.5, and 24 zeros. Each relative frequency is a fraction between zero and one, and when we add all 26 of them together we get 1.0. We begin by computing the relative frequencies of the 26 letters. But how should we calculate this score? We could just count the occurrences of e, but there is a better way.
Caesar cipher calculator crack#
We can exploit this fact to crack the Caesar cipher by scoring each of the 26 potential plaintexts for its likeness to English, based on letter frequencies. Most English writing uses all 26 letters, but it’s never a uniform distribution- e is far more common than z, for example. However, we’re going to use a different method: frequency analysis. This would work, since the true message should have by far the most English words in it. You could try each key and do a dictionary check on the results. The result of decrypting the message will almost certainly be gibberish for all but one key, but how can a computer recognize plausible English? We can easily do this by brute force, by trying all 26 possible keys. 1 Our goal is to crack a Caesar-encrypted message, which means to find its key, the rotation number used to encrypt it. There are only 26 Caesar ciphers on the other hand, there 26! possible letter substitution ciphers. The Caesar cipher is a special case of the substitution cipher, which maps all possible pieces of plaintext (usually single letters, but not always) to corresponding pieces of ciphertext. The exceptions are 0, which is pretty useless, and 13, which is ROT-13. Notice that with the Caesar cipher, we have to distinguish between encryption and decryption-one goes forwards and the other goes backwards. We only need to consider numbers between 0 and 25, since adding multiples of 26 makes no difference. The Caesar cipher generalizes this to numbers other than 13. The result of doing this to the plaintext (message) is called the ciphertext (coded message). You’ve probably heard of the ROT-13 cipher, where you rotate each letter around the alphabet by 13 positions. It’s significantly more interesting than “Hello, World!” but it only takes about a hundred lines to write-in fact, the line count by itself is a good indication of how expressive the language is. This problem is perfect for getting a sense of what it’s like to work in a given language. Whenever I play around with a new language, I always start by writing a program to crack a Caesar cipher.
Caesar cipher calculator how to#
Monday, 30 March 2015 Cracking the Caesar cipher How to crack a Caesar cipher using frequency analysis
